Pillow is a Python Imaging Library (PIL) fork. It is an open-source Python library used for image processing and manipulation. Pillow is a popular library among data scientists, computer vision researchers, and developers due to its flexibility and ease of use. It supports a variety of image formats including BMP, GIF, JPEG, PNG, TIFF, and WebP. In this article, we will learn how to read and process images using Pillow in Python.
Installation
Before we start, we need to install Pillow. You can install Pillow using pip by running the following command in your command prompt or terminal.
pip install Pillow
Once installed, we can use pillow to read and perform different operations on images.
Read Images using Pillow
To read an image using Pillow, we need to create an Image object. Pillow provides a function called Image.open()
to read an image. We just need to pass the image file path to this function. Let's see an example.
from PIL import Image
# Open an image file
image = Image.open("image.jpg")
# Display the image
image.show()
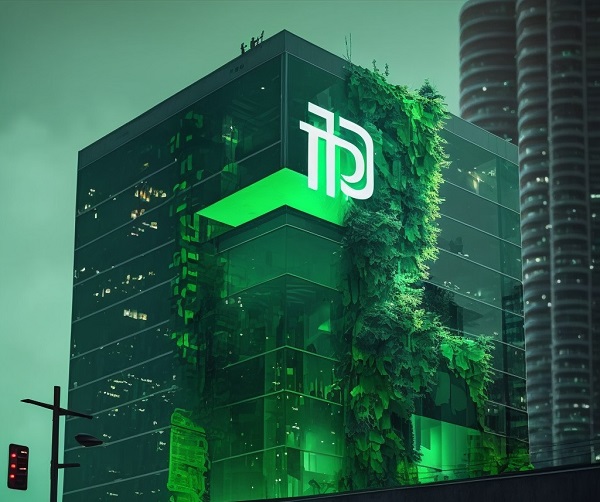
In the above example, we imported the Image module from the Pillow library. Then we used the Image.open()
function to open an image file named image.jpg
. Finally, we used the show()
function to display the image.
Process Images using Pillow
Pillow provides a wide range of functions to process and manipulate images. In this section, we will discuss some of the commonly used functions.
Resize Image
To resize an image using Pillow, we can use the resize()
function. This function takes a tuple of the new size as an argument. Let's see an example:
from PIL import Image
# Open an image file
image = Image.open("image.jpg")
# Resize the image
new_size = (500, 500)
image = image.resize(new_size)
# Display the image
image.show()
In the above example, we opened an image file named image.jpg
. Then we resized the image to a new size of (500, 500) using the resize function and show it.
Crop Image
To crop an image using Pillow, we can use the crop()
function. This function takes a tuple of the (left, upper, right, lower) pixel coordinates as an argument. Let's see an example:
from PIL import Image
# Open an image file
image = Image.open("image.jpg")
# Crop the image (left, upper, right, lower)
box = (100, 100, 400, 400)
image = image.crop(box)
# Display the image
image.show()
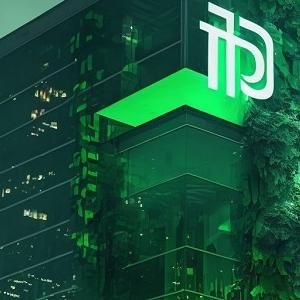
In the above example, we opened an image and then we cropped the image using the crop function. We specified the (left, upper, right, lower) pixel coordinates as (100, 100, 400, 400) to crop a rectangular region from the image and display the output image.
Convert Image to Grayscale
To convert an image to grayscale using Pillow, we can use the convert()
function. This function takes the string "L" as an argument to convert the image to grayscale. Let's see an example:
from PIL import Image
# Open an image file
image = Image.open("image.jpg")
# Convert the image to grayscale
image = image.convert("L")
# Display the image
image.show()
In above example, we convert image to grayscale using convert function and display output.
We can save final processed image using save()
function in pillow.
# save image to file
image.save("output.jpg")
In this article, we learned how to read and process images using Pillow in Python. We discussed how to resize an image, crop an image and convert an image to grayscale. Pillow provides many other functions for image processing and manipulation. You can refer to the Pillow documentation for more information on these functions. Pillow is a powerful library that can be used to build image-based applications and computer vision models.
For more information on pillow, check official Pillow documentation.
Comments (0)