Azure Blob Storage, a product from Microsoft's Azure suite, has emerged as a popular solution for unstructured data storage needs. From binary data, logs, backups, to massive datasets, this object storage service offers a flexible, cost-effective, and scalable way to hold and manage your data. This guide couples Azure Blob Storage's power with Python's simplicity, showing you how to conduct essential operations using the two together.
Prerequisites
Azure Account: You'll need an Azure subscription. If you don't have one, you can sign up for a free trial.
Python Environment: Ensure you have Python installed. This guide assumes you're familiar with basic Python programming.
Azure SDK for Python: Microsoft provides SDKs for various languages, and Python is no exception.
Now, once we are ready, we can start with Azure storage.
Setting Up Azure Blob Storage
Microsoft's Azure Portal offers an intuitive interface for managing all Azure resources, Blob Storage included:
- Begin by logging into the Azure Portal: https://portal.azure.com/
- Opt for "Create a resource".
- Use the search bar and type "Storage Account", selecting the option that appears.
- Hit "Create".
- Fill out the details. Make note of the resource group, location, and other configurations you choose. Click
Review + create
, followed by "Create" after the - system completes its validation checks.
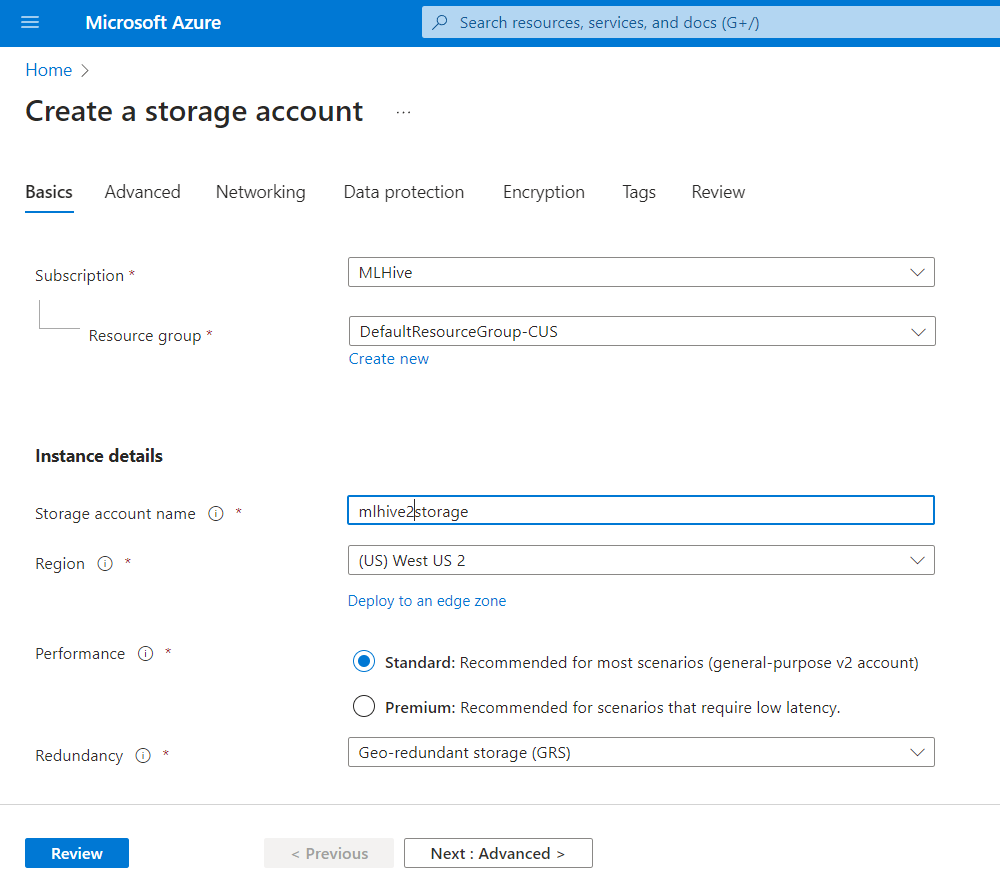
Extracting Access Keys
To connect your Python application securely to Blob Storage, you need access keys:
- Once your storage account creation completes, click on it from the dashboard.
- In the left sidebar, find and select “Access keys”.
- Two keys will appear. Copy either of them. This key will be the bridge between your Python code and Azure.
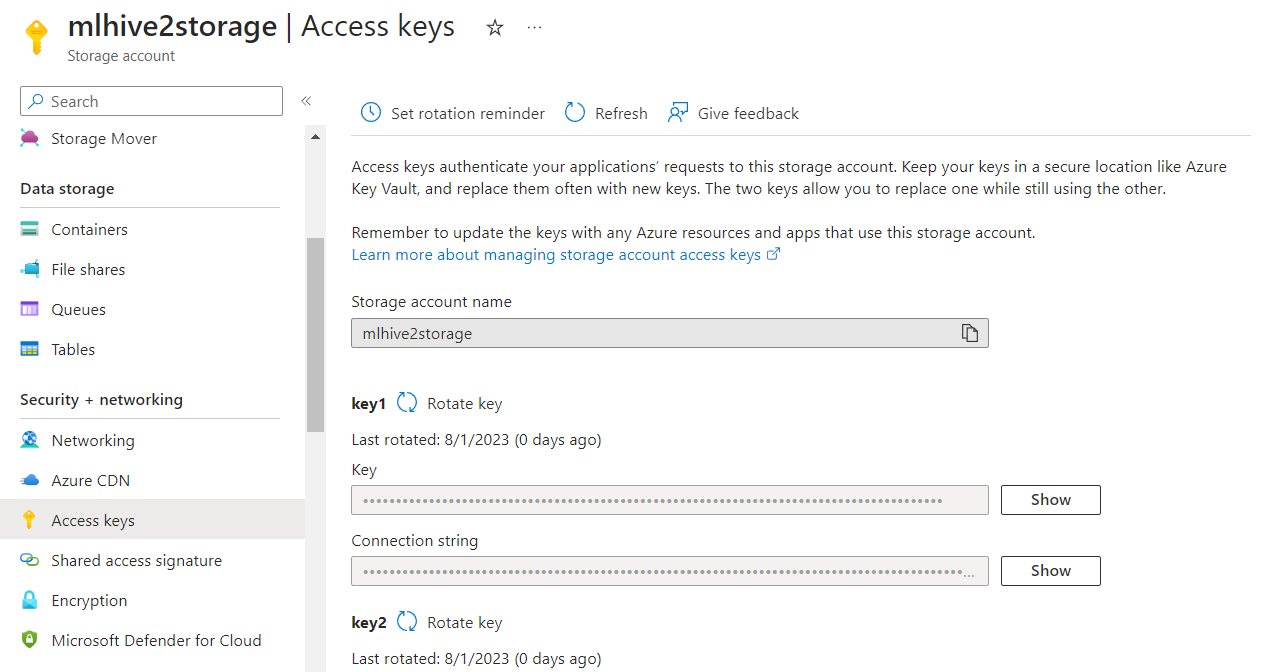
Python Interactions
With the SDK, Python can easily interact with Azure:
First, get the SDK with pip:
pip install azure-storage-blob
Then, script the initial connection:
from azure.storage.blob import BlobServiceClient
import os
connection_string = os.getenv("AZURE_CONNECTION", "YOUR_AZURE_CONNECTION_STRING")
blob_service_client = BlobServiceClient.from_connection_string(connection_string)
Replace the placeholder with your actual connection string.
Now, we can perform different actions using python like creating Blob Containers, Upload, Download and Delete files.
Creating a Container
container_name = "desired-container-name"
container_client = blob_service_client.create_container(container_name)
Once container is created, we can upload files to that container. Now, let's add a file (blob) to our container.
blob_name = "name_for_your_blob"
file_path = "local_path_to_your_file"
blob_client = blob_service_client.get_blob_client(container=container_name, blob=blob_name)
with open(file_path, "rb") as data:
blob_client.upload_blob(data)
Downloading Files
Fetching a blob is as straightforward as uploading.
storage_path = "files/filename"
download_location = "local_path_for_downloaded_file"
blob_client = blob_service_client.get_blob_client(blob=storage_path, container=container_name)
blob_data = blob_client.download_blob()
with open(download_location, "wb") as file:
blob_data.readinto(file)
Deleting Files
To remove a blob
# Path of file on storage, that you want to delete
storage_path = "files/filename"
blob_client = blob_service_client.get_blob_client(blob=storage_path, container=container_name)
blob_client.delete_blob()
Generating Access URLs
Sometimes, you might want to share a blob through a URL, often using a SAS (Shared Access Signature) token for limited access.
from azure.storage.blob import generate_blob_sas, BlobSasPermissions
sas_token = generate_blob_sas(
account_name="your-account-name",
container_name=container_name,
blob_name=blob_name,
account_key="your-access-key",
permission=BlobSasPermissions(read=True),
expiry=datetime.utcnow() + timedelta(hours=1)
)
shareable_url = f"https://{account_name}.blob.core.windows.net/{container_name}/{blob_name}?{sas_token}"
print(shareable_url)
Azure Blob Storage, combined with Python, provides a potent toolset for developers. Its flexibility in managing vast amounts of unstructured data, coupled with Python's widespread use in data-centric tasks, forms a powerful synergy. As we've demonstrated, starting with Blob Storage in Python is a seamless process, enabling rapid development and deployment of large-scale storage solutions.
Comments (0)