Barcode and QR Code systems are ubiquitous in everyday life. They are used for product identification, ticket validation, and much more. With OpenCV, it becomes relatively straightforward to develop a simple barcode and QR code scanner using Python. In this article, we'll delve deep into the methodology behind this, complemented with illustrative code examples.
Introduction
Barcode: A barcode represents data in varying space widths and parallel lines. Barcodes are 1D (one-dimensional) representations of data.
QR Code (Quick Response Code): A QR code is a two-dimensional barcode that can store more data than traditional barcodes. It consists of black squares arranged on a white grid.
Setting Up:
Before we begin, you need to have Python and OpenCV installed. Furthermore, to handle barcodes, we'll be using the pyzbar library. Install them using pip:
pip install opencv-python
pip install pyzbar
Barcode and QR Code Detection:
First, we get started by importing required packages in python:
import cv2
import numpy as np
from pyzbar.pyzbar import decode
Now, we can load images and decode them.
Reading and Decoding:
We'll first read the image, then use the decode function from pyzbar to find barcodes and QR codes.
def scan_image(image_path):
image = cv2.imread(image_path)
for barcode in decode(image):
my_data = barcode.data.decode('utf-8')
print(f"Detected: {my_data}")
# Draw a rectangle around the detected barcode or QR code
pts = barcode.polygon
if len(pts) == 4: # If a quadrilateral is found
pts2 = [[p.x, p.y] for p in barcode.polygon]
poly = cv2.polylines(image, [np.array(pts2)], True, (0, 255, 0), 2)
else:
rect = barcode.rect
cv2.rectangle(image, (rect.left, rect.top),
(rect.left + rect.width, rect.top + rect.height),
(0, 255, 0), 2)
# Put the data onto the image
cv2.putText(image, my_data, (pts[0].x, pts[0].y),
cv2.FONT_HERSHEY_SIMPLEX, 0.9, (0, 0, 255), 2)
cv2.imshow("Barcode/QR Code Scanner", image)
cv2.waitKey(0)
cv2.destroyAllWindows()
# Test the function
scan_image('path_to_your_image.jpg')
Check below input and output for a barcode and QR code image.
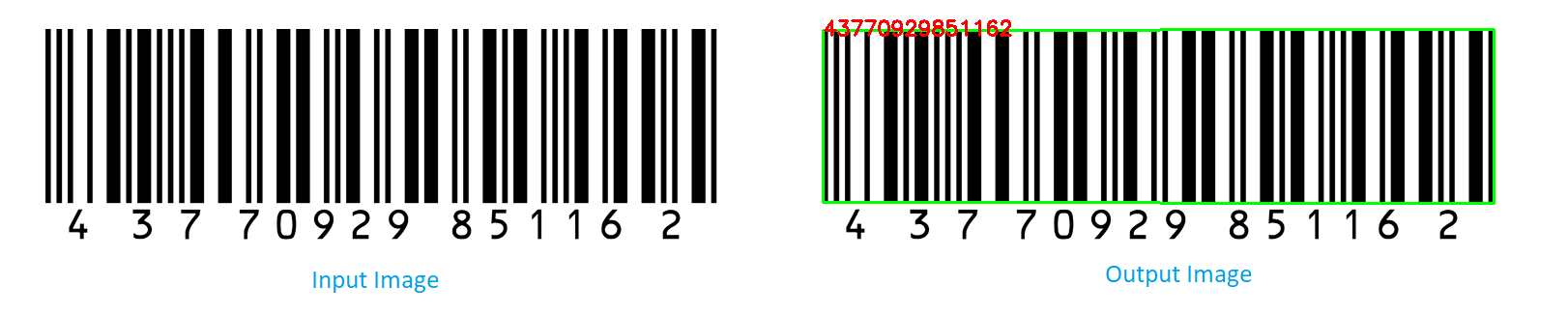
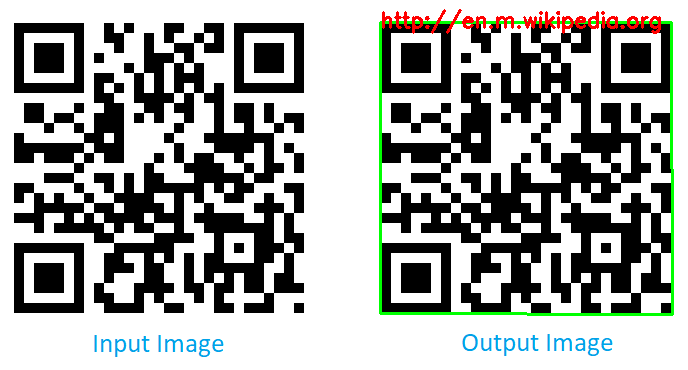
Notes:
-
Image Quality: The success of barcode and QR code detection heavily depends on the quality of the input image. Ensure it's clear and the codes aren't obscured or damaged.
-
Real-time Scanning: The code provided is for scanning static images. However, with minor modifications, you can easily use OpenCV's VideoCapture function to scan in real-time with a webcam.
-
Handling Multiple Codes:
pyzbar
can handle images with multiple barcodes and QR codes. The provided code will detect and annotate all of them. -
Data Types: Not all QR codes contain textual data. Some might have links, others might have other encoded data. Ensure your application can handle various types of data safely.
With OpenCV and the pyzbar library, building a barcode and QR code scanner in Python becomes an uncomplicated task. The scanner can be easily integrated into larger applications, enabling automated product identification, ticket validation, and much more.
Remember, while static image scanning serves as a good introduction, real-world applications often require real-time scanning capabilities. Challenge yourself by expanding the above code to work with live video streams and adding other features like sound notifications or integration with databases.
Comments (0)